How to use Oauth to get Authorization Code with Line Authentication
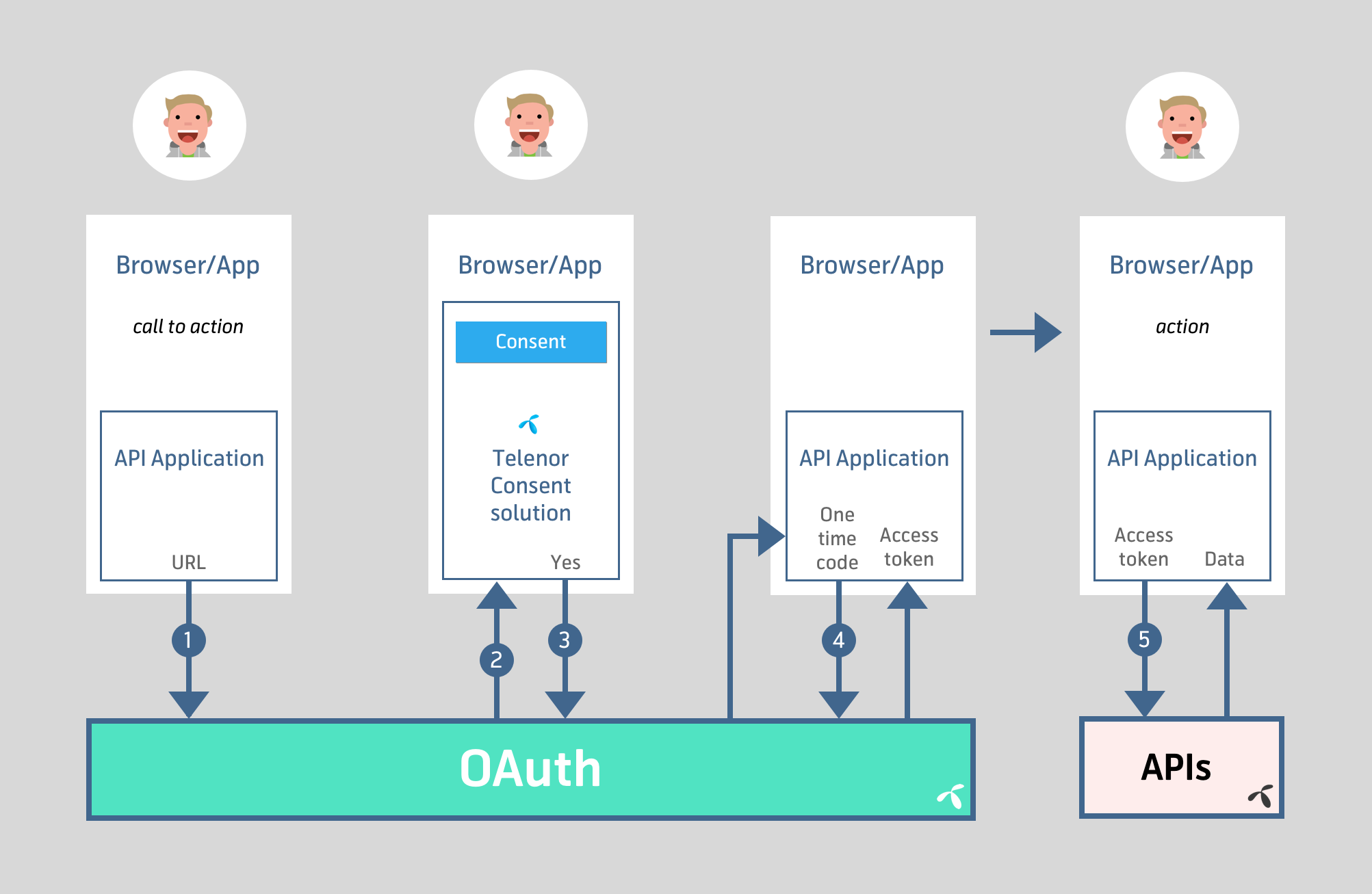
For APIs that require end user consent and authorization the OAuth flow above is required*. This is to ensure that applications don't access user data without proper authorization and without end-user consent.
Line Authentication means the user is automatically identified using the mobile network or by using an USSD-flow instead of the end-user entering a username/password.
To get access to the API the user must authorize your application by following a standard OAuth process *:
- End user wants to use functionality powered by Telenor in your application
- Your application redirects the user (or open a browser) to Telenor Norway using a client id (/oauth/v2/authorize?client_id=
&state= ) - End user is authenticated and gives consent so that your application can fetch the user's data
- Post a request to the OAuth-API (/oauth/v2/token) with the one time code returned in the callback (using client id and secret as basic authentication), to get an access token (time limited)
- Call Mobile User API to get data using the token
*) This figure, and the description, shows a best-case-situation. Several things might go wrong during the process. The most common will be that the end user doesn't give consent . This will also produce a callback to your application, but without an authorization code. Instead the following URL query parameters will hold information about the situation : error=access_denied & error_description=The resource owner denied the request. This error should be displayed to the end user in their browser in such a way that the user understands what happened, and what it means for the functionality in your application.
The parameters needed and where to get them is shown in the table below:
Name | What it's used for | Where to find it | Example values |
client_id | client_id_parameter is an OAuth parameter that identifies your client. Think of it as an application id. | Under your apps in your dashboard | xhdrs6uleK1xyZBO vX37PJ5wALcv1O9 |
client_secret | This is the secret for the client_id, which is an OAuth parameter that identifies your client. Think of it as an application password. | Under your apps in your dashboard | Glz2FV5XYOvAhFCE |
state | This is a random number that you set in your own application to avoid cross site scripting. You can also use it as a message id to match a callback request to your original request. | You can set it to anything you want, but preferably not a fixed value | 1234 |
Other | |||
end user consent | In this OAuth flow, to get an access token the end user will have to provide their consent, You, as a developer, will not need to know how this is done. You can try with your own subscription or user, but you will never be able to change how the consent is given as this is controlled by Telenor. | N/A | N/A |
Connectid username/password | These credentials are not involved in using the APIs at all. These are used by a developer to get access to the developer portal. This is a personal user that you can use for other Telenor services, like Min Sky. | If you need to register a new user, or if you've forgotten your password, you log in as a developer. If you need access to a specific company's apps, the administrator of that company must send you an invite to your connectid | username: myemailusername@example.com (or mobile number) Password: yourOwnChoice123 |
Min Bedrift Username/password | This user is not involved in the actual usage of the APIs. This is your single sign on user to the Min Bedrift Portal if your company has a Min Bedrift Agreement. You may have different roles within the company. If you are an API administrator, this is the user you should log in with to add developers. | If you need to register a new user, or if you've forgotten your password, you log in as a developer. If you need access to a specific company, a super administrator of that company must add you. | username: myemailusername Password: AbcDefG123 |
In short:
Tip:
It's highly recommended to use the state parameter to avoid cross site scripting. You can also use the state parameter as a message id to match the callback to your initial request.
Some pitfalls:
- For now, the network authentication flow requires the site to use http. So you should have a simple http page to initiate the flow. All other pages must be https. The ussd flow works on either protocols
- You must remember to configure the callback url for your app under “Applications” on the dashboard.
- If you're going to use the APIs from Javascript on your page, you must configure the CORS headers for your app under “Applications” on the dashboard.
- You can ignore the refresh_token and token_type response parameters in the doc. They are optional for now.
API Reference
oauth
Implementation notes
For use with OAuth 2.0 grant type authorization_code
For other grant types there is no need for issuing this request. Skip, and do the /oauth/v2/token request.
The first time the client application starts, it will need to get an access token.
The authorization flow is started by the client application opening a browser, either external or application embedded. Depending on what how what authorization mechanism the client is configured to use, the end user may now be redirected to a login page.The end user shall be able to verify that the login page is originating from a Telenor server by viewing the SSL certificate.Next, the browser accesses the authorize URL, and is given a redirect URL in return (link to the full spec for your reference ).The user agent should follow this redirect.
This request will respond with a 302 Found for a valid client_id, and redirect the end user to the authorization server.
Example invocation:"curl -w "%{redirect_url}
" https://api.telenor.no/oauth/v2/authorize?client_id=88....43" where the client_id is your Consumer Key
Example response for a valid client_id (302 Found): "https://identity.telenor.no/sso/login?samlRequest=fV....3D&authBadge=81....8c"
Open the authorize URL in a browser , e.g. "https://api.telenor.no/oauth/v2/authorize?client_id=88....4".
The user will be authenticated as required by the API Product. The API product documentation will describe which authentication mechanisms that are supported. For example by logging in with a valid username, password and in some cases also one time password(OTP) or other mechanisms like network authentication or ussd. Some also include end-user consent.
Regardless of authentication mechanism, if the process was successful, the service will redirect the browser to the callback uri configured for your application. Retrieve the code parameter from the URL "code=xxx" which will be the /oauth/v2/token request. You can now close the browser if you are in an app.
Once you have obtained the authorization code, the next step is to use this to obtain an access token by issuing a POST request to /oauth/v2/token.
If the process failed, or the user didn't authorize the application, an error code will be returned
NB: The "code" you obtain here is for single time use only using /oauth/v2/token and is only valid for a short period of time (minutes).
Parameters
-
client_id*
-
The client identifier, named Consumer Key on the developer portal
-
query
-
string
-
response_type
-
Value MUST be set to "code".
-
query
-
string
-
redirect_uri
-
If supplied, this MUST match the client callback uri defined for the client.
-
query
-
string
-
scope
-
The scope of the access request.
-
query
-
string
-
state
-
Recommended. Client provided state that can be used maintain state when receiving the callback to the client redirect_uri.
-
query
-
string
Error responses
- 400
- Error code 2 - Missing client_id queryparam
- 401
- Error code 1 - Invalid client id
- 403
- Error code 12 - Invalid RedirectURI
Error code 15 - Illegal Response Type
Error code 8 - Illegal or non authorized scope
- 500
- Error code 1 - Authbadge not generated
Error code 16 - Invalid App Attributes.Please contact Telenor Admin
Error code 2 - Internal Server Error
Implementation notes
Invalidate the access token.
The client makes a request to invalidate the access token.This is a optional operation.
Example invocation: "curl -v -X GET https://api.telenor.no/oauth/v2/logout --header "Authorization: Bearer OD....==" "
Example response (200 OK): OK!
Parameters
-
Authorization*
-
Authorization, Example: "Authorization: Bearer Xjh6f....MkjJH65
-
header
-
string
Error responses
- 401
- Error code 2 - Invalid access token
Error code 6 - Missing or bad Authorization header
- 500
- Error code 2 - Internal Server Error
Implementation notes
This token must be used to authorize all further API requests to the server, and is valid for a period of time.
- Authorization Code Grant
The authorization code grant type is used to obtain both access tokens and refresh tokens and is optimized for confidential clients. As a redirection-based flow, the client must be capable of interacting with the resource owner's user-agent (typically a web browser) and capable of receiving incoming requests (via redirection) from the authorization server.
Example invocation: "curl -v -X POST https://api.telenor.no/oauth/v2/token -u client_id:client_secret --data "code=5D7vaNS4" --data "grant_type=authorization_code" "
Example response (200 OK): "{"access_token" : "4P....uY", "expires_in" : 3599}", time in seconds.
NOTE:The authorization header is created from your client_id (Consumer Key) as your basic authentication username,and the client_secret (Consumer secret) as the basic authentication password. Basic authentication requires you to Base64 encode the combination of username:password, note: the colon separating the username and password and is in the form "Authorization: Basic OZXhhbXBsZWNsaWVudGlkOmV4YW1wbGVzZWNyZXQ="When using curl use can use '-u username:password' in order for curl to do the Base64 and add this header for you.
Parameters
-
Authorization*
-
Authorization, Basic authorization using the Consumer Key (client_id) as 'username' and Consumer secret (client_secret) as password. Base64 encode username:password. "Authorization: Basic QW....=="
Example: Authorization: Basic Q2xpZW50SWQ6U2VjcmV0 -
header
-
string
-
grant_type*
-
Grant type of token request.
Possible values: authorization_code, password, client_credentials -
formData
-
string
-
code*
-
Value of authorization code from get authorization call.
-
formData
-
string
-
scope
-
The scope of the access request.Use values returned in callback url after /authorize call.
-
formData
-
string
Response class (Status 200)
- access_tokenstring
- The token representing an authorization issued to the client and the logged in user
- expires_ininteger
- The lifetime in SECONDS of the AccessToken. E.g. a value of 3600 indicated that the access_token will expire in 1 hours from the time it was issued.
- token_typestring
- If returned, it will explicitly state the type of token issued. It will be ‘Bearer’ by default.
- refresh_tokenstring
- If returned, the refresh token can be used to obtain new access tokens using the authorization grant ‘refresh_token’. The refresh token itself will also be refreshed.
{ "access_token": "Iu25QXBwbGbljYXRpzQHRIuYlbGVub329XXQ3tOnMY3BRo0QyVFJUiN", "expires_in": "3600", "token_type": "Bearer", "refresh_token": "9XXQ3tOnMY3BRo0QyVFJUiNIu25QXBwbGbRIuYlbGVub32ljYXRpzQH" }
Error responses
- 400
- Error code 2 - Missing or invalid grant_type
Error code 3 - Missing code formparam
- 401
- Error code 1 - Invalid client id
Error code 21 - Unsufficient permissions to use requested grant_type
Error code 22 - Basic Authentication failed, bad username or password.
Error code 4 - Invalid authorization code
Error code 5 - Not authorized to create access token
Error code 6 - Missing or bad Authorization header
Error code 7 - Invalid refresh_token
- 403
- Error code 12 - Invalid RedirectURI
Error code 15 - Illegal Response Type
Error code 8 - Illegal or non authorized scope
- 500
- Error code 16 - Invalid App Attributes.Please contact Telenor Admin
Error code 2 - Internal Server Error